SPEEDO
Part I:
home>projects>speedo
part 1
I
recently completed a cycling expedition in Leh-Ladhak through
the YHAI
camp. But wait, this post is not about the expedition. You can
find the Flickr set
here.
I
hadn’t cycled for years and wanted to cycle again. But
suddenly heading for a high altitude (11500 feet) 240Km+ adventure
could have proved disastrous. The expedition was a perfect opportunity
to invest in a new cycle and reignite the lost passion. So a
month prior to the trip, I bought myself a Suncross mountain
bike and began practicing. It soon occurred to me that a speedo/odometer
could prove very handy. I simply couldn’t resist the temptation
of building one myself than to invest in an off-the-shelf solution.
But the month kept me busy in other things and the speedo project
could never see the light of day.
So
on my return, I took up the project and managed to make some
significant headway. The plan is to use a magnet-reed switch
pair to detect wheel revolutions, graphics LCD for displaying
the information and a PIC uC to do all the processing. I narrowed
down on the Nokia 3310 LCD (it’s easy to find/use, light
weight, cheap and there’s plenty on info available on
the web). For the uC, I chose the PIC18F452, 28pin microcontroller
(I had sampled many of them a while ago and were laying unused).
Although, economically, I think Atmega8 is a better option here.
Heres
the setup I plan to use in the final design:
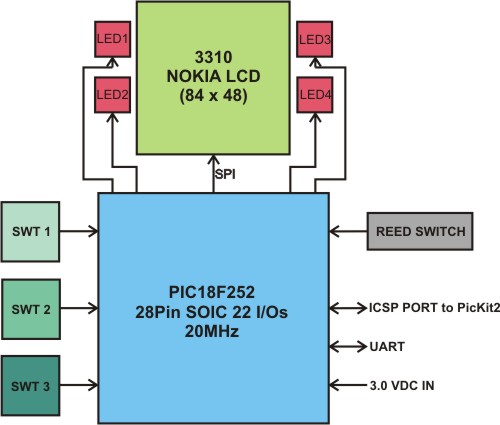
Note
that the LCD can only be powered by voltages from 2V to 3.3V.
Since the PIC can easily work from a 3V source, I plan to use
2xAAA cells. There is no inbuilt backlight on the Nokia LCD,
hence LED1 –LED4 are used for backlight and would also
serve well during debugging.
The
Nokia LCD is controlled via the SPI port. Till now I have managed
to build a decent library for it which can display characters
in normal/big fonts, display images and do the usual routines
like clearing, inverting, etc. I wanted to try out this LCD
for a long time and initially thought it might be difficult
to hack. But that’s certainly not the case. The control
is pretty straight forward once you have the SPI setup correctly.
I’ll write about the interface in-depth some time soon.
Heres
a schematic of the basic setup currently in place:
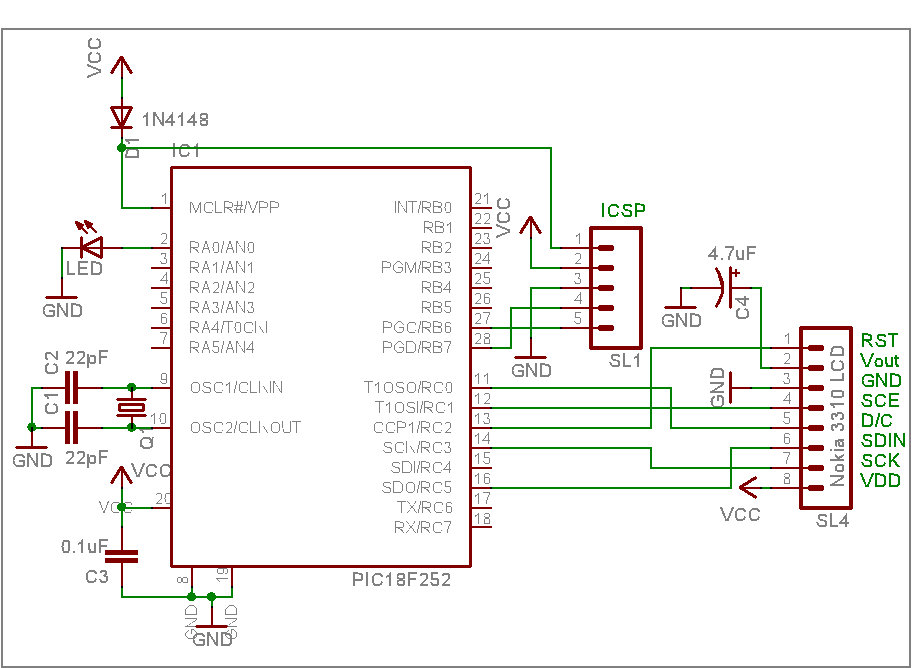
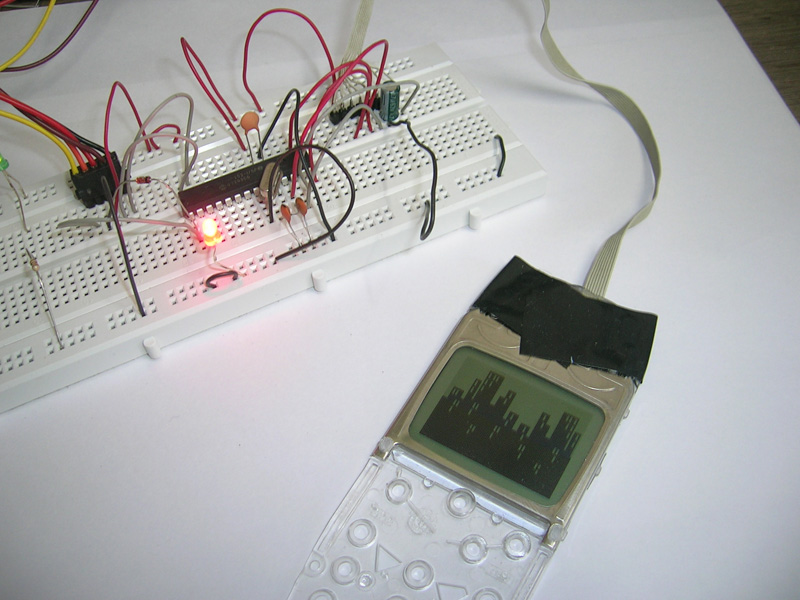
I
wrote a small script in Python that converts the bitmap (.bmp) images into nicely laid out
HEX numbers. I later simply copy-paste it into an array and
I’m good to go! The script makes use of the
Python Imaging Library (PIL) for reading the images.
The images can be designed in any photopaint application. They
have to be 1-bit black/white with a size of 84x48 pixels.
Heres
the script:
import Image
im = Image.open("sample.bmp")
x = 0
y = 0
z = 0
numL = 0
numH = 0
conv = [1,2,4,8]
while z<=5:
x = 0
while x<=83:
y = 0
while y<=3:
value = im.getpixel((x,y+(z*8)))
if value==0: numL = (numL | conv[y])
y += 1
while y<=7:
value = im.getpixel((x,y+(z*8)))
if value==0: numH = (numH | conv[y-4])
y += 1
print "0x"+('%x' %numH) + ('%x' %numL)+",",
numL = 0
numH = 0
x += 1
z += 1
Download the script: bitmap2hex.py
And the result is something like this:
0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0xf0, 0xfe, 0xfe, 0x0e, 0x0e, 0x0e, 0x0e,
0x0e, 0x0e, 0x0e, 0x0e, 0x0e, 0x0e, 0x00, 0x00, 0x00, 0x60,
0x70, 0x7c, 0x3c, 0x1e, 0x0e, 0x0e, 0x0e, 0x0e, 0x0e, 0x1c,
0xfc, 0xf8, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0xf0, 0xfe, 0xfe, 0x0e, 0x0e, 0x0e,
0x0e, 0x0e, 0x0e, 0x0e, 0x0e, 0x0e, 0x0e, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0e, 0x0f, 0x0f, 0x0d,
0x06, 0x07, 0x07, 0x07, 0x07, 0x07, 0x0f, 0x1e, 0xfc, 0xf8,
0xf0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0e,
0x0e, 0x0e, 0x0f, 0x1f, 0x3f, 0xf9, 0xf8, 0xe0, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0e, 0x0f, 0x0f,
0x0d, 0x06, 0x07, 0x07, 0x07, 0x07, 0x07, 0x0f, 0x1e, 0xfc,
0xf8, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x06, 0x1e, 0x3e, 0x3c, 0x78, 0x70, 0x70, 0x70, 0x70, 0x70,
0x38, 0x3c, 0x1f, 0x0f, 0x03, 0x00, 0x00, 0x06, 0x1e, 0x3e,
0x3c, 0x78, 0x70, 0x70, 0x70, 0x70, 0x70, 0x38, 0x3c, 0x1f,
0x0f, 0x03, 0x00, 0x00, 0x00, 0x70, 0x70, 0x70, 0x00, 0x00,
0x00, 0x06, 0x1e, 0x3e, 0x3c, 0x78, 0x70, 0x70, 0x70, 0x70,
0x70, 0x38, 0x3c, 0x1f, 0x0f, 0x03, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00
I
later plan build a simple GUI with additional functions and
which would directly churn out .c files.
Some
sample LCD screenshots:
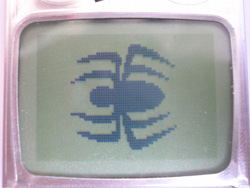
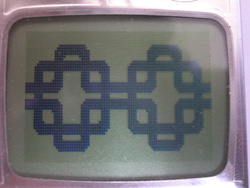
References:
1.
Sylvain Bissonnette's Website : He has written the nokiaLCD
driver for AVR microcontrollers.
2. Pete
Harrison: Pete uses a similar Nokia 3410 LCD (96x64 pixels)
for his micromouse. He has used a dsPIC microcontroller and
has published a library for the same.
I’ll
soon post the Part 2 of this project once I have the speedo
up and running on a PCB.
Last
updated on: 29 Sept 2008